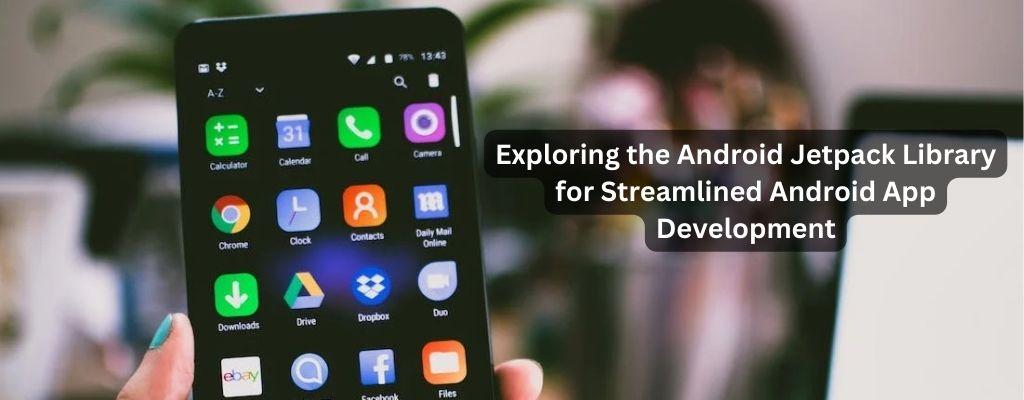
Android Jetpack Library is a comprehensive suite of tools and components provided by Google to streamline and enhance Android app development. The Android platform has been a trailblazer in the world of mobile operating systems for years. Its open-source nature, coupled with a massive user base and a thriving developer community, has enabled Android to continually evolve and set new standards in mobile app development. One of the most significant contributors to this evolution is the Android Jetpack library. Android Jetpack is not just a single library but a comprehensive suite of tools, components, and guidelines provided by Google to simplify and enhance Android app development. In this in-depth exploration, we will delve into the world of Android Jetpack, understanding its purpose, key components, and how it is revolutionizing the way developers create Android applications.
Table of Contents
ToggleUnderstanding Android Jetpack Library
Android Jetpack, launched by Google at the I/O conference in 2018, is not a standalone library, but rather an extensive collection of libraries, tools, and architectural guidance aimed at addressing common challenges faced by Android developers. It is designed to accelerate and streamline Android app development, allowing developers to focus on creating unique and innovative features instead of getting bogged down by repetitive, time-consuming tasks.
The primary objectives of Android Jetpack can be summarized as follows:
- Enhancing developer productivity: Jetpack provides a set of ready-to-use components that help developers write less code and achieve more. This reduces the development time and effort required to create feature-rich Android apps.
- Improving app quality: The components included in Jetpack are thoroughly tested, ensuring greater reliability and stability of apps. This means fewer bugs and crashes, resulting in a better user experience.
- Promoting best practices: Android Jetpack encourages developers to follow best practices for app architecture, UI design, and data management. This consistency across apps leads to more maintainable and understandable code.
- Enabling modern Android development: With the inclusion of Jetpack Compose, Android Jetpack library supports modern UI development, offering a declarative approach to creating user interfaces.
Key Components of Android Jetpack
Android Jetpack library consists of a wide range of components that are categorized into the following areas:
1. Foundation
- AppCompat: This component provides backward compatibility for modern Android features, ensuring apps work consistently across different Android versions.
- Android KTX: Kotlin extensions for Android that make Android development in Kotlin more concise and idiomatic.
- Multidex: Multidex support library, allowing applications to have multiple DEX files to overcome the 64K method reference limit.
2. Architecture
- ViewModel: ViewModel helps manage UI-related data. It survives configuration changes, allowing data to be retained during device rotation, for example.
- LiveData: LiveData is an observable data holder class that is lifecycle-aware, meaning it updates UI components only when the observed data is active and in a valid state.
- Room: Room is a persistence library that makes working with databases in Android much easier. It offers an abstraction layer over SQLite.
- WorkManager: WorkManager is a library that simplifies background work scheduling, allowing developers to execute deferrable and guaranteed background tasks.
3. UI
- Fragment: Fragments are essential building blocks of Android UI. Android Jetpack library simplifies fragment management, making dynamic and flexible user interfaces more accessible.
- Navigation: Navigation helps manage in-app navigation, offering a consistent and predictable user experience.
- Layout: Layout components offer tools for building consistent and flexible UIs, including ConstraintLayout, ViewPager2, and more.
4. Behavior
- Notifications: This component simplifies the process of creating and displaying notifications to users, enhancing user engagement.
- Permissions: Managing app permissions is a critical aspect of Android development. Jetpack makes this process more straightforward.
- Sharing: Sharing content between apps is a common task. Android Jetpack library provides a sharing component to make it easy to share data.
5. Data Binding
- Data Binding: Data Binding allows you to bind UI components in your layouts to data sources in your app using a declarative format.
6. Jetpack Compose
- Jetpack Compose: Jetpack Compose is a modern Android UI toolkit that uses a declarative approach to build user interfaces. It is a significant departure from the traditional XML-based UI development, offering a more intuitive and efficient way to create UIs.
The architecture components of Android Jetpack, including ViewModel, LiveData, Room, and WorkManager, play a crucial role in simplifying the development of robust and maintainable Android applications.
Streamlining Android App Development with Android Jetpack
Android Jetpack library has several ways in which it streamlines the app development process. Let’s explore these aspects in detail:
1. Reducing Boilerplate Code
Boilerplate code refers to the repetitive, low-value code that developers often have to write to accomplish common tasks. Android Jetpack, particularly its architecture components, helps reduce the amount of boilerplate code developers need to write. This results in more concise and readable code, which is easier to maintain.
Take, for example, the ViewModel component. It is responsible for managing UI-related data and ensuring that this data survives configuration changes, such as when the device is rotated. In traditional Android development, handling these situations required writing a considerable amount of boilerplate code. With ViewModel, this complexity is abstracted away, making it easier to manage data between different components of the app.
2. Ensuring Consistency
One of the fundamental principles of Android Jetpack is to encourage best practices and ensure consistency across apps. When developers use Jetpack’s architecture components like ViewModel and LiveData, they follow recommended patterns for data management and app structure. This not only results in more reliable apps but also makes it easier for other developers to understand and work on the code.
Consistency also extends to other aspects of app development. For instance, the Navigation component simplifies in-app navigation, reducing the complexity of managing navigation flows. By defining navigation paths and transitions in a centralized location, Jetpack ensures a consistent user experience.
3. Simplifying UI Development
Jetpack Compose is a significant leap forward in terms of simplifying UI development. Instead of using XML layout files, developers can create UI elements using a concise, Kotlin-based DSL. This declarative approach makes it easier to visualize the structure of the interface and create complex, responsive layouts with less effort.
Traditionally, creating user interfaces in Android involved a mix of XML layout files and Java or Kotlin code. This approach often led to verbose and complex layout files, making it challenging to maintain and modify UIs. With Jetpack Compose, UI elements are defined in a more intuitive and declarative way. This not only results in cleaner code but also makes it easier to design and implement user interfaces.
4. Enhancing Testing
Testing is an essential aspect of app development. Android Jetpack’s architecture components are designed to be testable. For example, the ViewModel, LiveData, and Room components can be easily tested using unit tests. This ensures that critical app logic and data management work as expected.
Testing is particularly crucial for complex apps, as it helps identify and fix issues early in the development process. By providing testable components, Jetpack simplifies the testing process, leading to more robust and reliable apps.
5. Easing Navigation
App navigation is a common source of complexity in Android app development. Managing the flow of screens and user interactions can be challenging, especially in apps with multiple screens and complex navigation requirements. The Navigation component of Jetpack simplifies in-app navigation by providing a structured way to define and manage navigation paths.
Traditionally, handling navigation involved various techniques, often resulting in tangled and hard-to-maintain code. With the Navigation component, developers can define navigation destinations, actions, and transitions in a dedicated navigation graph. This approach makes it easier to understand and modify the app’s navigation flow.
Getting Started with Android Jetpack
If you’re eager to embrace Android Jetpack and leverage its benefits for your Android app development projects, here’s how you can get started:
1. Set Up Android Studio
Android Jetpack is fully supported in Android Studio, the official integrated development environment (IDE) for Android app development. Make sure you have Android Studio installed and updated to the latest version to take full advantage of Jetpack.
2. Create a New Project
You can start a new Android project using Android Jetpack, or if you have an existing project, you can integrate Jetpack components as needed.
3. Add Dependencies
To incorporate Jetpack components into your project, you need to add the required dependencies in your app’s Gradle build files. Jetpack components are typically available through the AndroidX library, which is an Android extension library for Jetpack components. You can easily add these dependencies by specifying them in your Gradle files.
4. Learn and Experiment
Android Jetpack components come with extensive documentation, code samples, and tutorials. It’s essential to invest time in understanding how each component works and experimenting with them in your projects. Google’s official documentation and online tutorials can be valuable resources for learning Jetpack.
5. Stay Updated
Android Jetpack is an evolving set of libraries and tools. To make the most of its capabilities, it’s essential to stay updated with the latest developments. Google regularly releases updates, introduces new components, and enhances existing ones to align with modern Android development practices.
Conclusion
Android Jetpack is a game-changer in the world of Android app development. It streamlines the development process, making it more efficient and productive for developers. By reducing boilerplate code, ensuring consistency, simplifying UI development, enhancing testing, and easing navigation, Android Jetpack empowers developers to create high-quality Android apps more easily than ever before.
If you’re an Android app developer, there’s no better time to explore Android Jetpack and incorporate its powerful components into your projects. By doing so, you can take your app development to the next level and provide a better user experience for your audience. Android Jetpack is not just a set of libraries; it’s a pathway to a brighter and more efficient future for Android app development.